1. Let’s start by creating a new WordPress and Laravel integration from WordPress Pete.
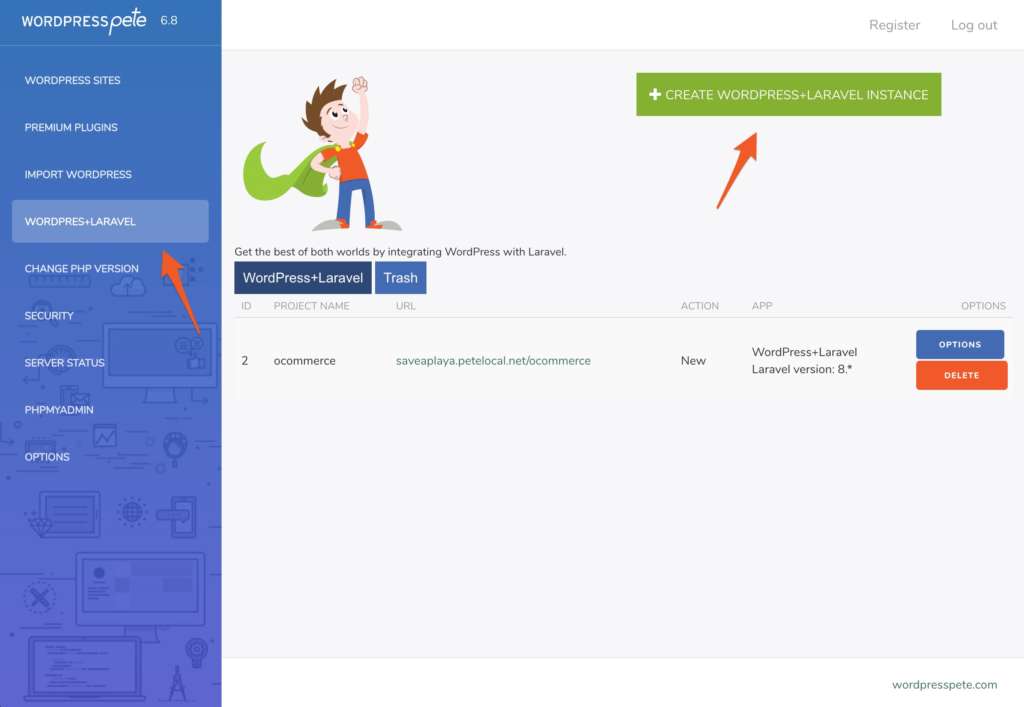
2. For this tutorial, we will use Laravel 8, and the name of our Laravel app will be woodashboard. You must have previously imported a WordPress site.
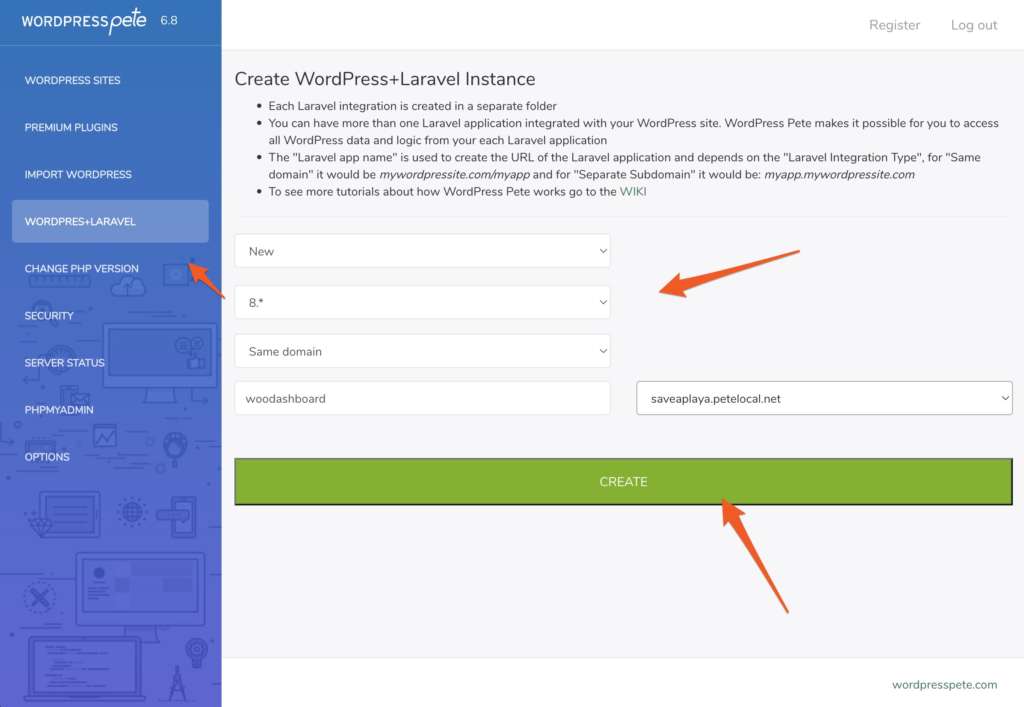
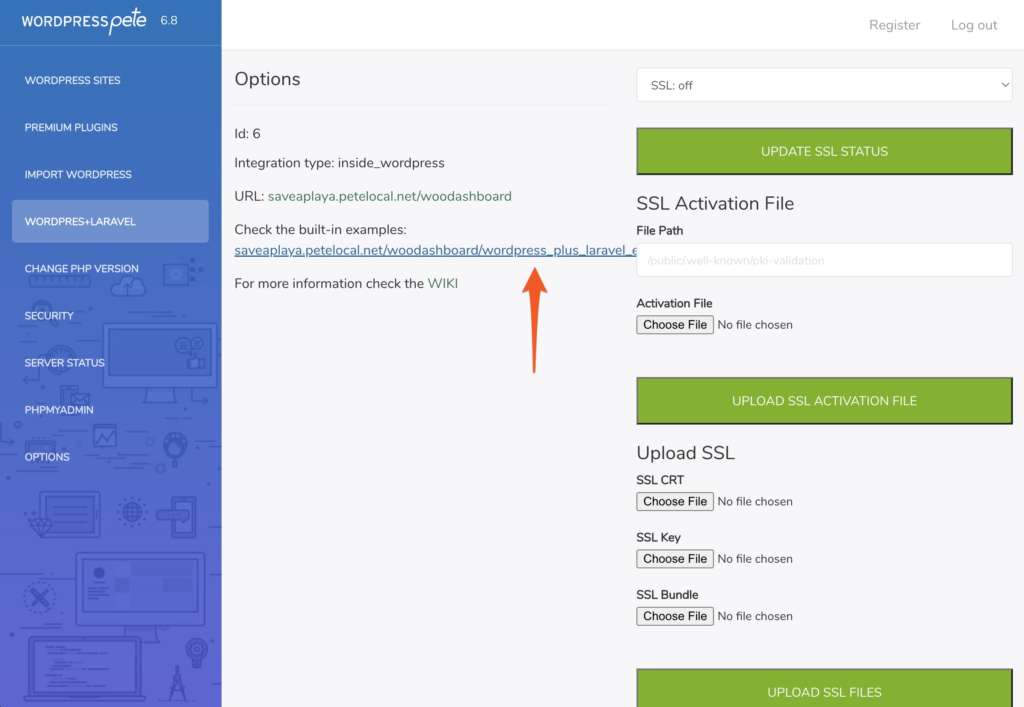
3. We can verify everything is OK by clicking on the link to see the examples.
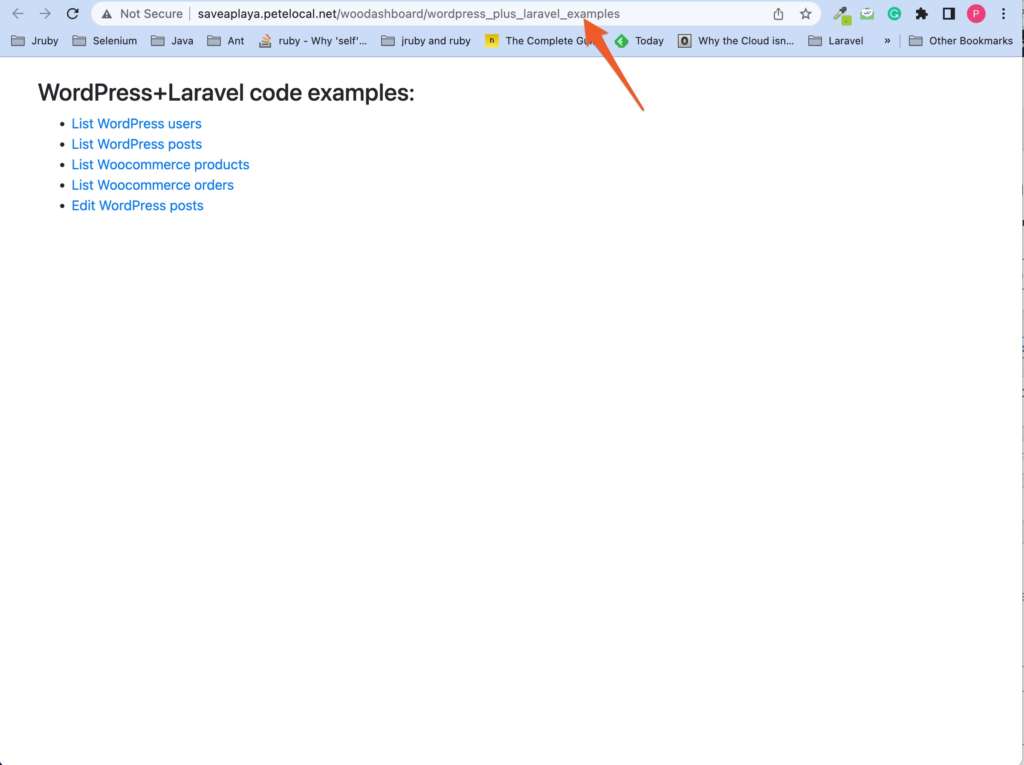
4. After this, it is a good idea to familiarize yourself with the structure of how your files look. Open the WordPress Pete installation folder with your favorite text editor. Since I am old school, I use TextMate.
- The WordPress Pete installation folder name is saveaplaya
- The name of the WordPress site folder is saveaplayapetelocalnet
- The name of the Laravel app integrated into WordPress is woodashboard
- Inside woodasboard you can find all the files of the Laravel app.
The integration Pete does of WordPress and Laravel is clean. That means all WordPress and Laravel files are complete, and you can update each app to its latest versions without breaking the integration. Cool right?
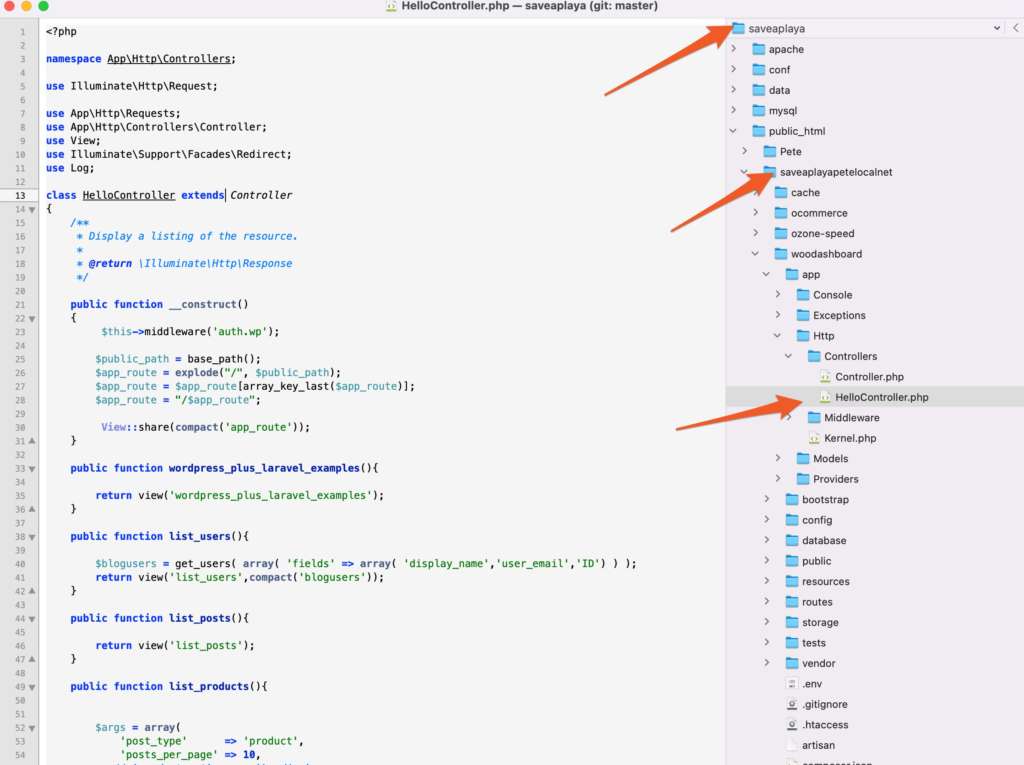
5. To create a controller in Laravel from the terminal, you must enter the apache container in docker. For this, go to the installation guide of your operating system and copy the command to enter the apache container in docker.
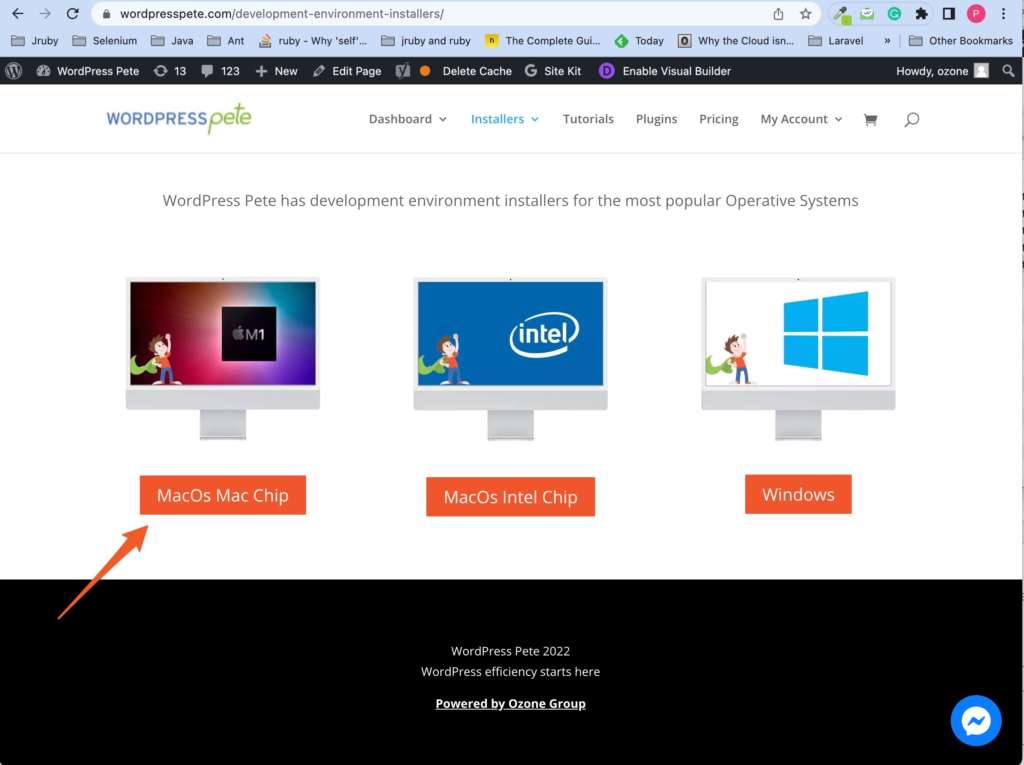
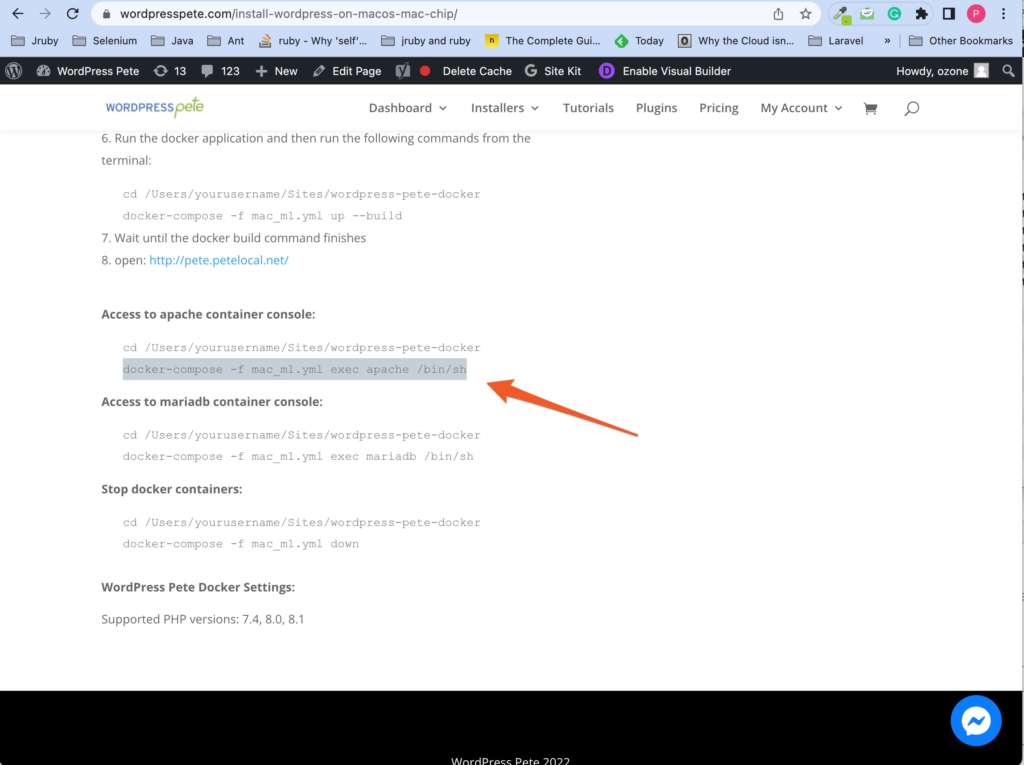
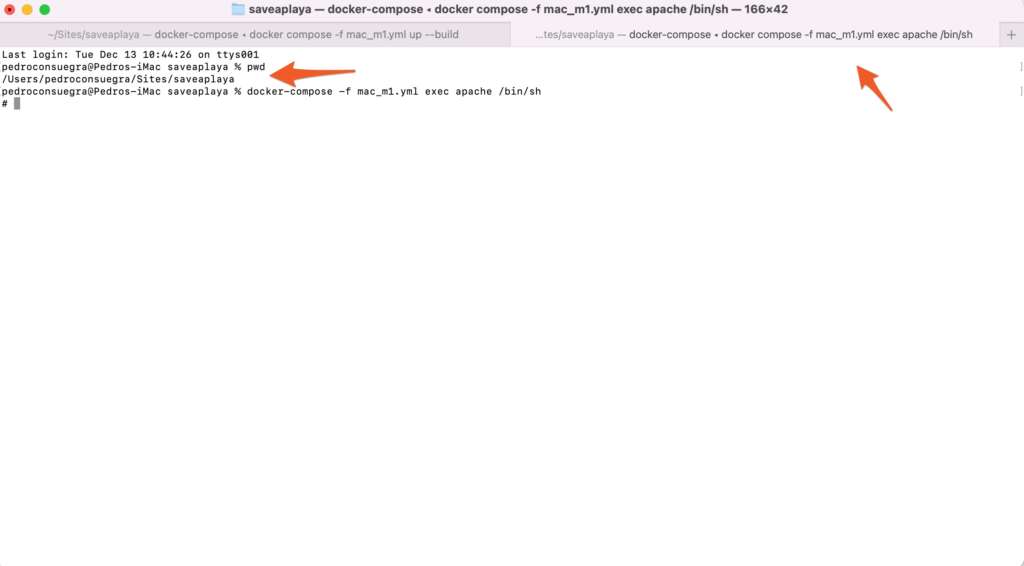
6. Now, browse the Laravel app folder and run the command to create a controller.
php artisan make:controller WooDashboard
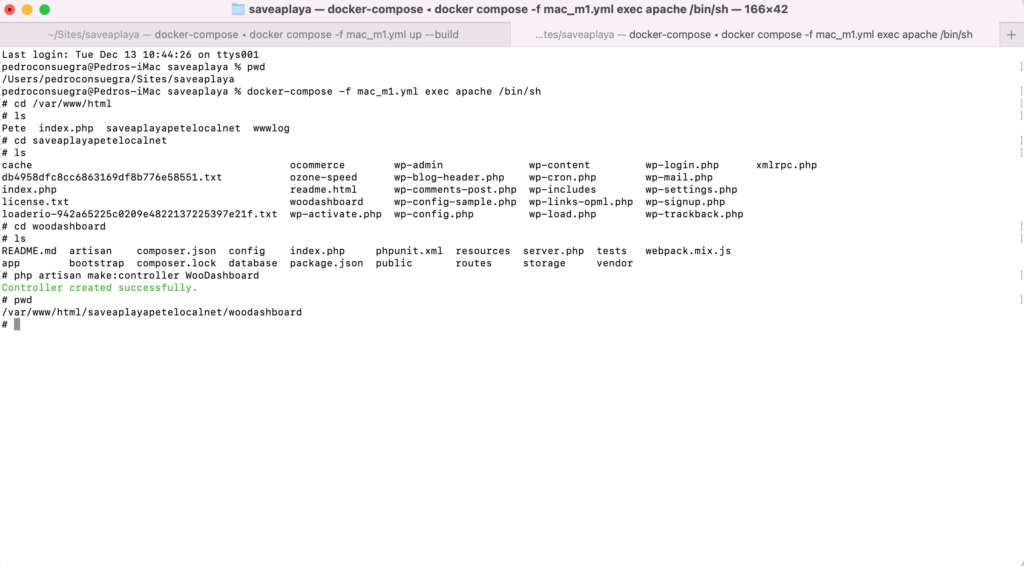
After this, we copy and paste this code into our WooDashboard controller.
woodashboard/app/Http/Controllers/WooDashboard.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
//add this
use Illuminate\Support\Facades\Config;
class WooDashboard extends Controller
{
//add this
public function orders(){
$db_config = Config::get('database.connections.'.Config::get('database.default'));
$mysqli = new \mysqli($db_config["host"], $db_config["username"], $db_config["password"], $db_config["database"]);
if ($mysqli->connect_errno) {
echo "Failed to connect to MySQL: (" . $mysqli->connect_errno . ") " . $mysqli->connect_error;
}
$query = "select
p.ID as order_id,
p.post_date,
max( CASE WHEN pm.meta_key = '_billing_email' and p.ID = pm.post_id THEN pm.meta_value END ) as billing_email,
max( CASE WHEN pm.meta_key = '_billing_first_name' and p.ID = pm.post_id THEN pm.meta_value END ) as _billing_first_name,
max( CASE WHEN pm.meta_key = '_billing_last_name' and p.ID = pm.post_id THEN pm.meta_value END ) as _billing_last_name,
max( CASE WHEN pm.meta_key = '_billing_address_1' and p.ID = pm.post_id THEN pm.meta_value END ) as _billing_address_1,
max( CASE WHEN pm.meta_key = '_billing_address_2' and p.ID = pm.post_id THEN pm.meta_value END ) as _billing_address_2,
max( CASE WHEN pm.meta_key = '_billing_city' and p.ID = pm.post_id THEN pm.meta_value END ) as _billing_city,
max( CASE WHEN pm.meta_key = '_billing_state' and p.ID = pm.post_id THEN pm.meta_value END ) as _billing_state,
max( CASE WHEN pm.meta_key = '_billing_postcode' and p.ID = pm.post_id THEN pm.meta_value END ) as _billing_postcode,
max( CASE WHEN pm.meta_key = '_shipping_first_name' and p.ID = pm.post_id THEN pm.meta_value END ) as _shipping_first_name,
max( CASE WHEN pm.meta_key = '_shipping_last_name' and p.ID = pm.post_id THEN pm.meta_value END ) as _shipping_last_name,
max( CASE WHEN pm.meta_key = '_shipping_address_1' and p.ID = pm.post_id THEN pm.meta_value END ) as _shipping_address_1,
max( CASE WHEN pm.meta_key = '_shipping_address_2' and p.ID = pm.post_id THEN pm.meta_value END ) as _shipping_address_2,
max( CASE WHEN pm.meta_key = '_shipping_city' and p.ID = pm.post_id THEN pm.meta_value END ) as _shipping_city,
max( CASE WHEN pm.meta_key = '_shipping_state' and p.ID = pm.post_id THEN pm.meta_value END ) as _shipping_state,
max( CASE WHEN pm.meta_key = '_shipping_postcode' and p.ID = pm.post_id THEN pm.meta_value END ) as _shipping_postcode,
max( CASE WHEN pm.meta_key = '_order_total' and p.ID = pm.post_id THEN pm.meta_value END ) as order_total,
max( CASE WHEN pm.meta_key = '_order_tax' and p.ID = pm.post_id THEN pm.meta_value END ) as order_tax,
max( CASE WHEN pm.meta_key = '_paid_date' and p.ID = pm.post_id THEN pm.meta_value END ) as paid_date,
max( CASE WHEN pm.meta_key = '_customer_user' and p.ID = pm.post_id THEN pm.meta_value END ) as customer_user,
( select group_concat( order_item_name separator '|' ) from wp_woocommerce_order_items where order_id = p.ID ) as order_items
from
wp_posts p
join wp_postmeta pm on p.ID = pm.post_id
join wp_woocommerce_order_items oi on p.ID = oi.order_id
where
p.post_type = 'shop_order' and
p.post_date BETWEEN '2022-10-01' AND '2022-12-31' and
p.post_status = 'wc-completed'
group by
p.ID";
$result = $mysqli->query($query);
return view('WooDashboard.orders',compact('result'));
}
}
7. Now we create the Laravel view
woodashboard/resources/views/WooDashboard/orders.blade.php
<html>
<head>
<!-- CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.5.3/dist/css/bootstrap.min.css" integrity="sha384-TX8t27EcRE3e/ihU7zmQxVncDAy5uIKz4rEkgIXeMed4M0jlfIDPvg6uqKI2xXr2" crossorigin="anonymous">
<!-- jQuery and JS bundle w/ Popper.js -->
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@4.5.3/dist/js/bootstrap.bundle.min.js" integrity="sha384-ho+j7jyWK8fNQe+A12Hb8AhRq26LrZ/JpcUGGOn+Y7RsweNrtN/tE3MoK7ZeZDyx" crossorigin="anonymous"></script>
</head>
<body>
<div class="container">
<br />
<h3>All completed orders</h3>
<table class="table table-hover">
<thead>
<tr>
<th>order_id</th>
<th>billing_first_name</th>
<th>billing_email</th>
<th>order_total</th>
</tr>
</thead>
<tbody>
@while($row = $result->fetch_assoc())
<tr>
<td>{{$row["order_id"]}}</td>
<td>{{$row["_billing_first_name"]}}</td>
<td>{{$row["billing_email"]}}</td>
<td>{{$row["order_total"]}}</td>
</tr>
@endwhile
</tbody>
</table>
</div>
</body>
</html>
8. Now we declare the route for this action
woodashboard/routes/web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\HelloController;
//add this
use App\Http\Controllers\WooDashboard;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Route::get('list_users', [HelloController::class,'list_users']);
Route::get('list_orders', [HelloController::class,'list_orders']);
Route::get('list_posts', [HelloController::class,'list_posts']);
Route::get('list_products', [HelloController::class,'list_products']);
Route::get('edit_posts', [HelloController::class, 'edit_posts']);
Route::get('edit_post', [HelloController::class, 'edit_post']);
Route::post('update_post', [HelloController::class, 'update_post']);
Route::get('/wordpress_plus_laravel_examples', [HelloController::class, 'wordpress_plus_laravel_examples']);
//add this
Route::get('orders', [WooDashboard::class, 'orders']);
9. Now we can see the list of woocommerce orders from Laravel
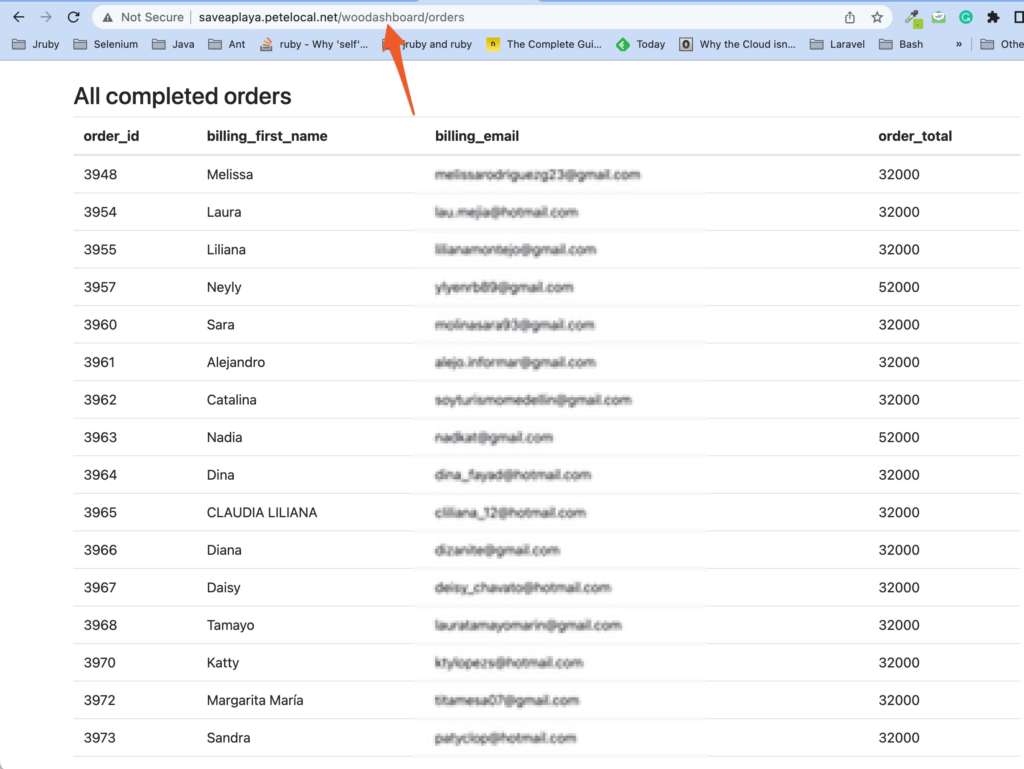